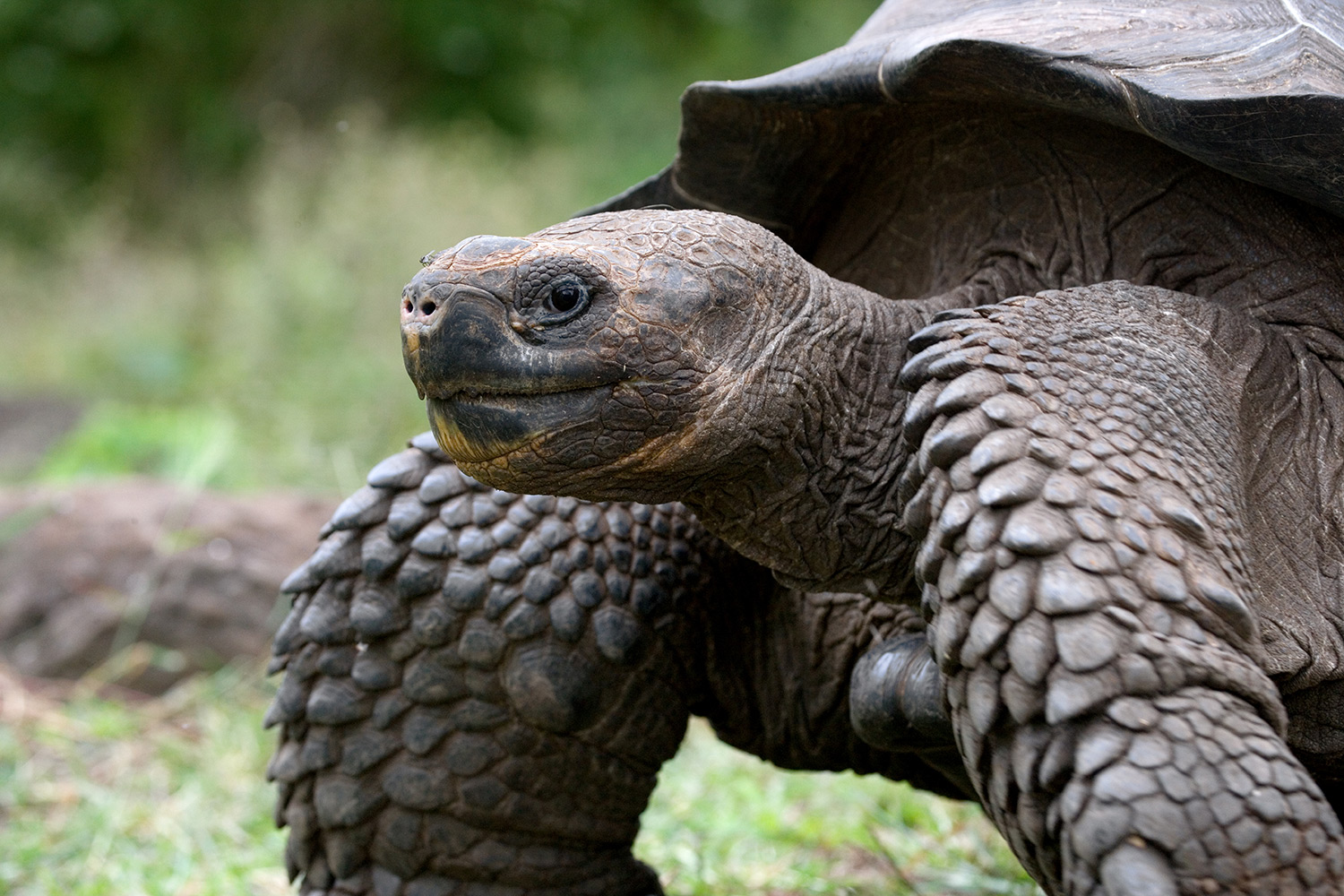
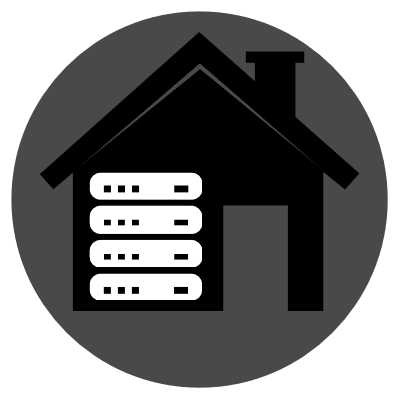
Ah I see you mentioned the cuts are only a few seconds long. This wouldn’t catch that very well.
If you have a server outside of your network you could simply hold open a TCP connection and report when it breaks, but I’ll admit at that point it’s outside of what I’ve had to deal with.
You must have the distinct privilege of not living in the USA or several other Western countries.
If you mean jump ship off that ISP, there's nothing you can do. You can go to another ISP (if there even is one in your area), who will do the exact same thing. You can jump ship entirely and not have internet, I guess.